目次
1. はじめに
C言語は、組み込みシステムやシステムプログラミングなど、幅広い用途で使われるプログラミング言語です。その中でも、「データ型」と「サイズ」は、プログラムのメモリ使用やパフォーマンスに大きな影響を与えます。特に、異なる環境(32bit / 64bit、Windows / Linux など)でプログラムを実行する場合、データ型のサイズが変わることがあるため、正しい型を選択することが重要です。
本記事では、C言語の基本的なデータ型のサイズ、環境による違い、適切な型選びのポイントについて解説します。さらに、sizeof
演算子の使い方や、固定幅整数型(stdint.h
)の活用方法など、実践的な情報も紹介します。これにより、C言語の型に関する理解を深め、効率的なプログラミングを行えるようになるでしょう。
2. C言語の基本データ型とサイズ
2.1 整数型のサイズ一覧
データ型 | 32bit環境 | 64bit環境 | 最小サイズ |
---|---|---|---|
char | 1バイト | 1バイト | 1バイト |
short | 2バイト | 2バイト | 2バイト |
int | 4バイト | 4バイト | 2バイト |
long | 4バイト | 8バイト | 4バイト |
long long | 8バイト | 8バイト | 8バイト |
2.2 浮動小数点型のサイズ一覧
データ型 | 32bit環境 | 64bit環境 |
---|---|---|
float | 4バイト | 4バイト |
double | 8バイト | 8バイト |
long double | 8~16バイト | 16バイト |
3. データ型サイズの環境依存性
3.1 OSやコンパイラごとのサイズの違い
データ型 | Windows (LLP64) | Linux (LP64) | 32bit 環境 |
---|---|---|---|
int | 4バイト | 4バイト | 4バイト |
long | 4バイト | 8バイト | 4バイト |
long long | 8バイト | 8バイト | 8バイト |
4. sizeof
演算子でデータ型のサイズを確認
4.1 sizeof
の基本
#include <stdio.h>
int main() {
printf("Size of int: %zu bytes\n", sizeof(int));
printf("Size of double: %zu bytes\n", sizeof(double));
return 0;
}
5. 固定幅整数型(stdint.h
の活用)
#include <stdio.h>
#include <stdint.h>
int main() {
int8_t a = 100;
uint16_t b = 50000;
int32_t c = -123456;
uint64_t d = 1000000000ULL;
printf("Size of int8_t: %zu bytes\n", sizeof(a));
printf("Size of uint16_t: %zu bytes\n", sizeof(b));
printf("Size of int32_t: %zu bytes\n", sizeof(c));
printf("Size of uint64_t: %zu bytes\n", sizeof(d));
return 0;
}
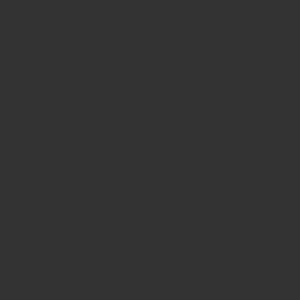
6. 構造体のサイズとアライメント
6.1 構造体のメモリレイアウト
#include <stdio.h>
struct example {
char a;
int b;
double c;
};
int main() {
printf("Size of struct: %zu bytes\n", sizeof(struct example));
return 0;
}
7. 適切なデータ型の選び方
7.1 整数型の選び方
データ型 | サイズ | 範囲 |
---|---|---|
int8_t | 1B | -128 ~ 127 |
uint8_t | 1B | 0 ~ 255 |
int32_t | 4B | 約 -2.1B ~ 2.1B |
uint32_t | 4B | 0 ~ 4.2B |
8. FAQ(よくある質問)
Q1: sizeof
の結果が unsigned int
なのはなぜですか?
A:
C言語では、sizeof
の戻り値は size_t
型であり、符号なし整数型です。負のサイズが存在しないため、安全な型として定義されています。
size_t size = sizeof(int);
printf("Size of int: %zu bytes\n", size);
9. まとめ
9.1 この記事の重要ポイント
✅ データ型のサイズは環境依存
✅ sizeof
を使って実際のサイズを確認
✅ 固定幅整数型を活用する
✅ 構造体のサイズはメモリアライメントに影響される
✅ 適切なデータ型の選び方